Understanding Client Components and Client Boundaries in Next.js: The Role of the "use client" Directive
Next.js has revolutionized how we build React applications by introducing powerful features that allow for better server-side rendering (SSR) and static site generation (SSG). With the introduction of React Server Components (RSC), Next.js further optimizes rendering performance by separating server-rendered and client-rendered code. One key concept to grasp when working with Next.js and React Server Components is the "use client" directive and its relationship to client components and client boundaries.
In this blog post, we'll explore how these elements work together in Next.js, what they mean from an architectural standpoint, and how they impact the way we build modern applications.
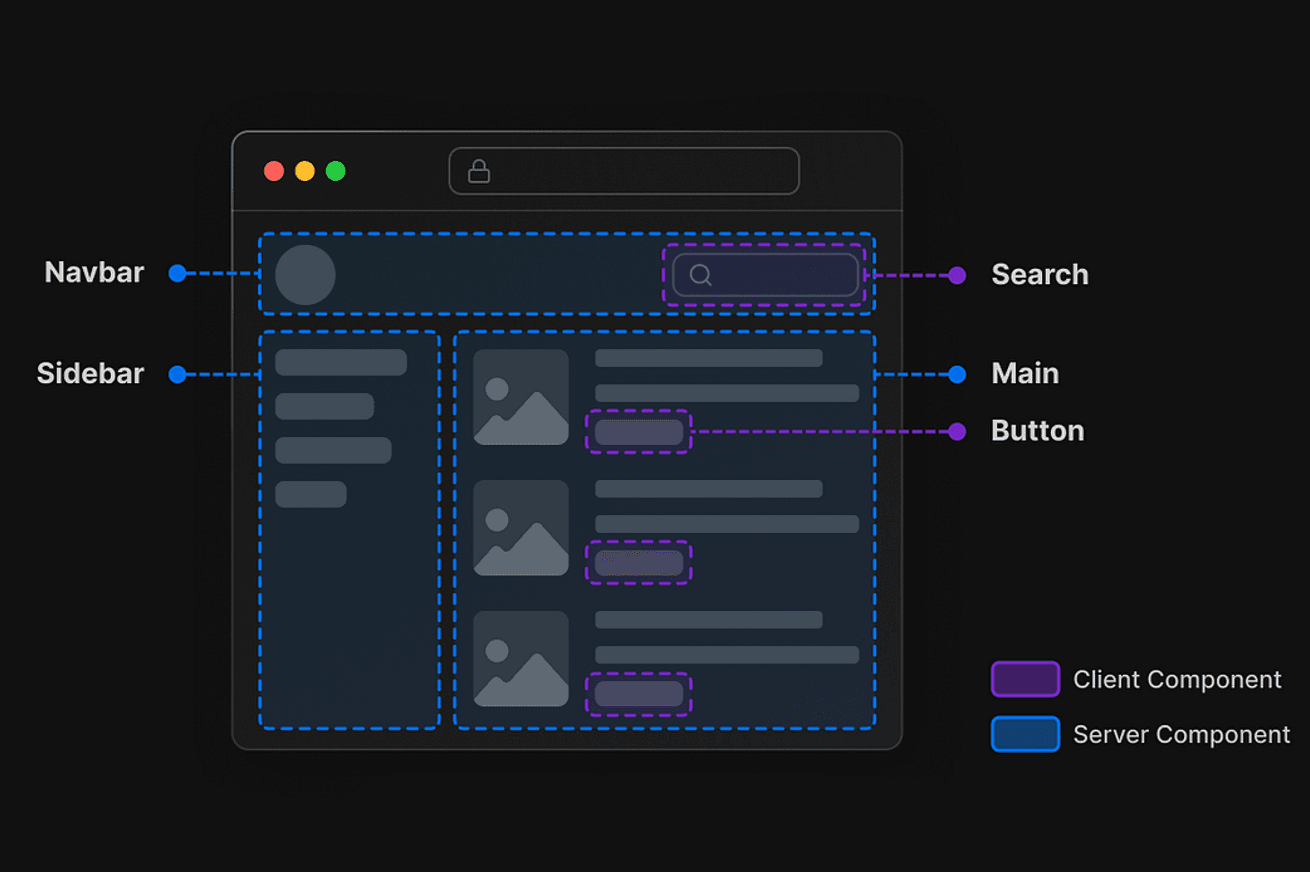
What is the "use client" Directive?
The "use client" directive is a special annotation in Next.js that tells the framework which components should run on the client-side. By default, components in Next.js are server-side rendered (SSR), meaning they are executed and rendered on the server before being sent to the client. However, there are times when you need components to behave dynamically or rely on client-side features such as state, effects, or event listeners.
The "use client" directive marks a component as a client component, which will be executed in the browser. This is crucial when working with React Server Components (RSC), as they are intended to run on the server and render only static HTML. The "use client" directive breaks that server-side rendering, signaling that the component should be hydrated on the client after the initial page load.
// Example of a client component using the "use client" directive
'use client';
import React, { useState } from 'react';
export default function ClientCounter() {
const [count, setCount] = useState(0);
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
<p>Count: {count}</p>
</div>
);
}
In this example, the ClientCounter
component is wrapped with the "use client" directive, making it run on the client-side. Without this directive, Next.js would try to render the component on the server, which would break due to the use of useState
, a client-side React hook.
Client Boundaries vs Client Components
In Next.js, the client boundary is a concept introduced alongside React Server Components. It defines the line where server-rendered code stops, and client-rendered code begins. A client component is a component that is rendered on the client side, often with interactive behavior or dynamic data fetching.
However, a client boundary is not the same as a client component. While a client component is typically responsible for interactive elements like buttons, forms, or client-specific state, a client boundary is a special construct that ensures that no server-rendered data is passed to the client component inappropriately.
A client boundary is necessary to define what parts of your app should be rendered on the server and which ones should be rendered on the client. It is a structural boundary that Next.js uses to differentiate between server-side rendering and client-side rendering.
Here’s where it gets interesting: Client boundaries can only accept non-serializable data as props. This means that you cannot pass functions, promises, or other non-serializable data (such as Date
objects) directly from a server component to a client component. Instead, you must create a client component using the "use client" directive, and from the client boundary component you can pass non-serializable data as props.
The "Gotcha": Passing Functions Between Server and Client Components
One of the most confusing aspects of working with React Server Components (RSC) and Next.js is the restriction on passing functions from a server component to a client component. Since functions are not serializable, trying to pass them directly from a server-rendered component to a client-rendered one will result in an error.
Example of Invalid Server-to-Client Function Passing:
// Server component
export default function ServerComponent() {
const handleClick = () => alert('Button clicked');
return (
<ClientComponent onClick={handleClick} />
);
}
// Client component - will throw an error because onClick is a function
'use client';
function ClientComponent({ onClick }) {
return <button onClick={onClick}>Click Me</button>;
}
The solution to this limitation is to have your server component render your client boundary component and then have your client boundary component pass any non serializable data as props to its child component.
Correct Way: Wrapping a Client Component in a Client Boundary
// Server component
export default function ServerComponent() {
return <ClientBoundary />;
}
// Client boundary component - renders the client component
'use client';
function ClientBoundary() {
const handleClick = () => alert('Button clicked');
return <ClientComponent onClick={handleClick} />;
}
// Client component - this will work
function ClientComponent({ onClick }) {
return <button onClick={onClick}>Click Me</button>;
}
In this corrected example, ClientBoundary is a client boundary that renders ClientComponent. And client component accepts the handleClick function as a prop. By marking the boundary component with "use client", we are able to pass functions from the client boundary component to the client components.
Why Client Boundaries are Important
The concept of client boundaries is fundamental when structuring Next.js apps, especially when working with React Server Components. Client boundaries help delineate areas of your application that require server-side rendering from those that need client-side interactivity.
This distinction is important for several reasons:
-
Performance optimization: By clearly separating server-rendered components from client-rendered ones, Next.js can optimize the rendering process, making sure that only the necessary data is fetched from the server while allowing client-side components to handle interactivity.
-
Architectural clarity: Understanding where your app’s boundaries lie—between server-rendered pages and client-rendered interactivity—helps you structure your components more effectively. Instead of mixing server logic with client logic, you can clearly define which parts of your app should handle state and interactions and which parts should focus on data fetching and rendering.
-
Avoiding unnecessary complexity: By recognizing the need for client boundaries and the restriction on passing functions, developers are encouraged to rethink how they architect their apps. This results in a more deliberate and modular design where each component has a clear responsibility.
Conclusion
The introduction of React Server Components and the "use client" directive in Next.js marks a significant shift in how we build and architect React applications. The distinction between client components and client boundaries allows us to optimize our apps by defining clear boundaries between server-rendered and client-rendered code.
By embracing client boundaries and understanding the limitations of passing functions and non-serializable data, developers can create more efficient, modular, and maintainable applications. As Next.js continues to evolve, mastering these concepts will become crucial for building scalable and performant web applications in the future.
In the end, the careful separation of server-side and client-side concerns in Next.js offers a new paradigm that brings clarity to how modern React apps should be structured—one that both developers and employers will appreciate as they look to improve performance and maintainability in their applications.